3 min to read
C# Singleton Pattern
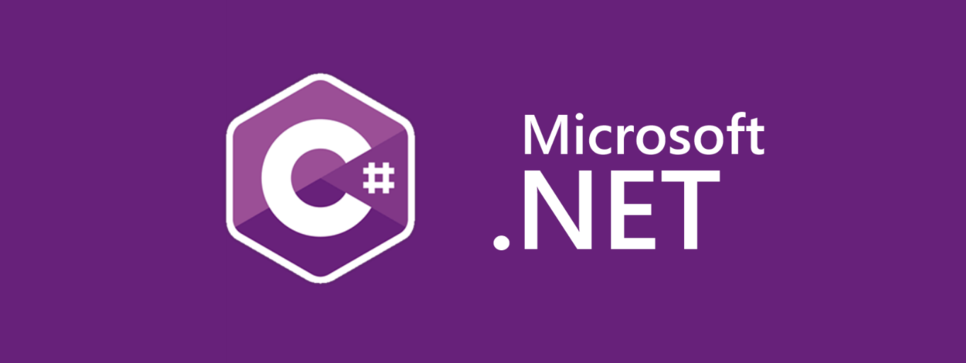
Singleton Pattern π€
-
μ±κΈν€μ μ ν리μΌμ΄μ μ΄ μ€ν λ μ΄ν λ¨ νκ°μ μΈμ€ν΄μ€λ§μ μμ±νκ³ μ ν λ μ¬μ©νλ ν¨ν΄μ΄λ€.
-
ννκ² μ°μ΄λ λμμΈ ν¨ν΄μ΄λ©°, μ£Όλ‘ νκ²½μ€μ κ³Ό κ°μ΄ νλλ‘ κ΄λ¦¬ν λ μ¬μ©νλ€.
-
C#μμλ μ±κΈν€μ ꡬννλ λ°©λ²μ΄ μ¬λ¬κ°μ§κ° μλλ° μ΄λ² ν¬μ€νΈμμ νμΈν΄λ³΄μ.
1 - UnsafeSingleton
- κΈ°λ³Έμ μΌλ‘ λ€μκ³Ό κ°μ΄ μ±κΈν€μ ꡬνν μ μλλ° μ΄λ κ² κ΅¬ννκ² λλ©΄ λ©ν°μ€λ λ νκ²½μμ λ¬Έμ κ° λ°μνλ€.
λ κ° μ΄μμ μ€λ λμμ μΈμ€ν΄μ€μ μ κ·Όμ ν λ κ°κΈ° λ€λ₯Έ μΈμ€ν΄μ€κ° μμ±λ μ μκΈ° λλ¬Έμ μ±κΈν€μ μλ°λλ νμκ° λ°μνλ€.
class UnsafeSingleton : Singleton
{
private static UnsafeSingleton _instance;
public static UnsafeSingleton Instance
{
get
{
if (_instance is null)
{
_instance = new UnsafeSingleton();
}
return _instance;
}
}
protected UnsafeSingleton() => Console.WriteLine("Create UnsafeSingleton instance!!");
}
2 - SafeSingleton
- 1λ²μ μ€λ λμ μμ νμ§ μμΌλ―λ‘ μ€λ λμ μμ νκ² κ΅¬ννλ©΄ 2λ²κ³Ό κ°λ€.
νμ§λ§ 2λ²μλ λ¬Έμ κ° μμΌλ.. μΈμ€ν΄μ€λ₯Ό μ°Έμ‘°ν λ λ§λ€ lockμΌλ‘ μΈν΄ μ±λ₯ μ νκ° λ°μν μ μλ€.
class SafeSingleton : Singleton
{
private static object _thislock = new object();
private static SafeSingleton _instance;
public static SafeSingleton Instance
{
get
{
lock (_thislock)
{
if (_instance is null)
{
_instance = new SafeSingleton();
}
}
return _instance;
}
}
protected SafeSingleton() => Console.WriteLine("Create SafeSingleton instance!!");
}
3 - DCLSingleton
- 2λ²μ lockμΌλ‘ μΈν μ±λ₯μ νλ₯Ό λ§κ³ μ λμ¨ λ°©λ²μ΄ Double Checked Lockμ΄λ€.
//Double Checked Locking
class DCLSingleton : Singleton
{
private static object _thislock = new object();
private static DCLSingleton _instance;
public static DCLSingleton Instance
{
get
{
if (_instance is null)
{
lock (_thislock)
{
if (_instance is null)
{
_instance = new DCLSingleton();
}
}
}
return _instance;
}
}
protected DCLSingleton() => Console.WriteLine("Create DCLSingleton instance!!");
}
4 - StaticSingleton
- static constructorμ μ΄μ©νμ¬ κ΅¬νν μλ μλ€.
class StaticSingleton : Singleton
{
private static readonly StaticSingleton _instance;
static StaticSingleton()
{
_instance = new StaticSingleton();
}
public static StaticSingleton Instance => _instance;
protected StaticSingleton() => Console.WriteLine("Create StaticSingleton instance!!");
}
5 - LazySingleton
- Lazyλ₯Ό μ΄μ©νμ¬ κ΅¬νν μλ μλλ°, Lazyλ μ¦μ μμ±μ΄ μλ μ κ·Όνλ € ν λ μμ±μ΄ λλ€.
class LazySingleton : Singleton
{
private static readonly Lazy<LazySingleton> _instance = new Lazy<LazySingleton>(() => new LazySingleton());
public static LazySingleton Instance => _instance.Value;
protected LazySingleton() => Console.WriteLine("Create LazySingleton instance!!");
}