4 min to read
C# ZipArchive
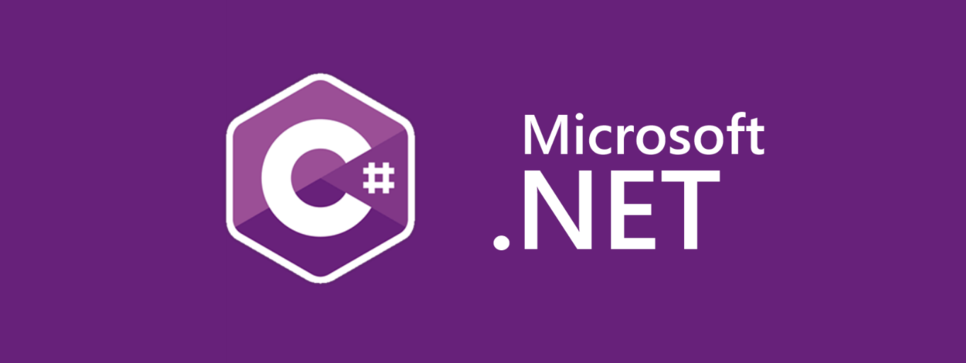
ZipArchive ๐
- ์ฐธ์กฐ
- System.IO.Compression
- System.IO.Compression.FileSystem
.Net Framework 4.5 ๋ถํฐ ์์ถ์ ๋ํ ๋ผ์ด๋ธ๋ฌ๋ฆฌ๊ฐ ์ ๊ณต์ด ๋๋ฉฐ ์ด๋ฒ ํฌ์คํธ์์๋ ์ ๊ณต๋๋ ๋ผ์ด๋ธ๋ฌ๋ฆฌ ์ค ZipArchive๋ฅผ ์ฌ์ฉํ๋ค.
- ์์ถํ๊ธฐ
internal static void CreateZip(string contentsFolderPath, string saveZipPath, string extention = "*") { if (!Directory.Exists(contentsFolderPath)) throw new DirectoryNotFoundException($"The directory is not found. \n{contentsFolderPath}"); if (!Directory.Exists(saveZipPath)) Directory.CreateDirectory(Path.GetDirectoryName(saveZipPath)); using (var fs = new FileStream(saveZipPath, FileMode.Create, FileAccess.ReadWrite)) using (var za = new ZipArchive(fs, ZipArchiveMode.Create)) { foreach (var filePath in Directory.EnumerateFiles(contentsFolderPath, $"*.{extention}", SearchOption.AllDirectories)) { try { za.CreateEntryFromFile(filePath, Path.GetFileName(filePath)); //var entry = za.CreateEntry(Path.GetFileName(filePath)); //using (var es = entry.Open()) //using (var writer = new StreamWriter(es)) //{ // writer.Write(File.ReadAllText(filePath)); //} } catch { throw; } } } }
- ZipArchive ํด๋์ค์ ํ์ฅ์ธ๋จธ๋์ธ CreateEntryFromFile ์ ์
// ์์ฝ: // ์์ถ zip ๋ณด๊ด ํ์ผ์ ์ถ๊ฐ ํ ์ฌ ํ์ผ์ ๋ณด๊ด ํฉ๋๋ค. // // ๋งค๊ฐ ๋ณ์: // destination: // Zip ๋ณด๊ด ํ์ผ์ ์ถ๊ฐ ํฉ๋๋ค. // // sourceFileName: // ๋ณด๊ด ํ์ผ์ ๋ ํ ๊ฒฝ๋ก์ ๋๋ค. ์๋ ๊ฒฝ๋ก ๋๋ ์ ๋ ๊ฒฝ๋ก๋ฅผ ์ง์ ํ ์ ์์ต๋๋ค. ์๋ ๊ฒฝ๋ก๋ ํ์ฌ ์์ ๋๋ ํฐ๋ฆฌ์ ์๋์ ์ผ๋ก ํด์๋ฉ๋๋ค. // // entryName: // Zip ๋ณด๊ด ํ์ผ์์ ๋ง๋ค ํญ๋ชฉ์ ์ด๋ฆ์ ๋๋ค. // // ๋ฐํ ๊ฐ: // Zip ๋ณด๊ด ํ์ผ์์ ์ ํญ๋ชฉ์ ๋ ํ ๋ํผ. // public static ZipArchiveEntry CreateEntryFromFile(this ZipArchive destination, string sourceFileName, string entryName);
- sourceFileName์ contents๋ฅผ ๊ฐ์ ธ์ entryName์ ์ด๋ฆ์ผ๋ก ZipArchive entry์ ์ถ๊ฐํ๋ ๊ฒ ๊ฐ๋ค. (์ฃผ์ ๊ตฌ๋ฌธ๊ณผ ๋์ผํ ๊ธฐ๋ฅ์ธ ๋ฏ ํ๋ค.)
- ์ฃผ์๊ตฌ๋ฌธ์ ZipArchive ํด๋์ค์ ์ธ์คํด์ค ๋ฉ์๋์ธ CreateEntry๋ฅผ ์ฌ์ฉํ๊ฒ๋๋ฉด
๋น
ํญ๋ชฉ์ entry๊ฐ ์์ฑ๋๋ฏ๋ก ํด๋น entry์ stream์ ๊ฐ์ ธ์ ์ฃผ์๊ตฌ๋ฌธ์ฒ๋ผ write ํด์ฃผ์ด์ผํ๋ค. (์ถ๊ฐ์ ์ธ write ์์ด ํด๋น ํ์ผ์ contents๋ง ๊ฐ์ ธ์ค๋ ๊ฒฝ์ฐ๋ฉด CreateEntryFromFile๋ฅผ ์ฌ์ฉํ๋ฉด ๋ ๊ฒ ๊ฐ๋ค.)
- ZipArchive ํด๋์ค์ ํ์ฅ์ธ๋จธ๋์ธ CreateEntryFromFile ์ ์
- ์์ถ ํด์ ํ๊ธฐ
internal static void ExtractZip(string zipPath, string extractFolderPath) { if (!File.Exists(zipPath)) throw new FileNotFoundException($"The file is not found. \n{zipPath}"); if (!Directory.Exists(extractFolderPath)) Directory.CreateDirectory(Path.GetDirectoryName(extractFolderPath)); using (var zipArchive = ZipFile.OpenRead(zipPath)) { foreach (var zipArchiveEntry in zipArchive.Entries) { try { string folderPath = Path.GetDirectoryName(Path.Combine(extractFolderPath, zipArchiveEntry.FullName)); if (!Directory.Exists(folderPath)) Directory.CreateDirectory(folderPath); zipArchiveEntry.ExtractToFile(Path.Combine(extractFolderPath, zipArchiveEntry.FullName)); } catch { throw; } } } }
- ์์ถ ํด์ ๋ ๊ฐ๋จํ๊ฒ zipํ์ผ์ ๊ฒฝ๋ก๋ฅผ ZipArchive๋ก ์ฝ์ด๋ค์ฌ์ ์์ ์๋ ํญ๋ชฉ(entry)๋ฅผ ์ํํ๋ฉฐ ํ์ฅ๋ฉ์๋์ธ ExtractToFile๋ฅผ ์ด์ฉํ์ฌ ์ง์ ๊ฒฝ๋ก์ ํ์ผ์ ์ด๋ค.
- ์ฌ์ฉ
static void Main(string[] args) { var contentsPath = @"Sample"; var zipPath = Path.Combine(contentsPath, "sample.zip"); Zip.CreateZip(contentsPath, zipPath, "txt"); Zip.ExtractZip(zipPath, Path.Combine(contentsPath, "zipFolder")); }
-
์ํ
- ๊ฒฐ๊ณผ